Object List
Often, you need to group objects with a common property or type, or with a common goal in the game. For example, you need to group the enemy objects, the pickable items, the platform objects, so you can test if the player collides with them.
For this purpose, the Scene Editor allows grouping the objects of the scene into a JavaScript array.
You can create an Object List using by dropping a List built-in block on the scene.
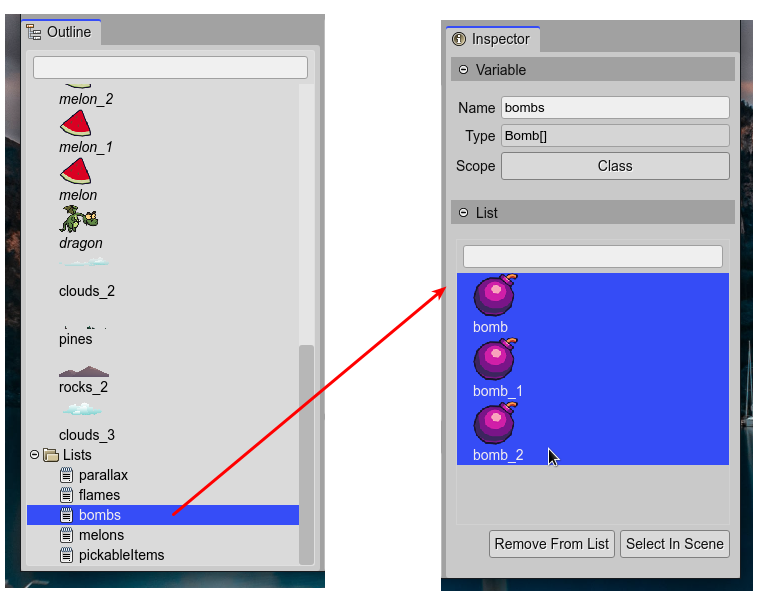
To add an object to an Object List, you can set the Lists property of the object, in the Inspector view.
The code generated by the scene compiler, to create a list, is like this:
this.pickableItems = [melon, melon_1, melon_2, bomb, bomb_1, bomb_2];
If the output language is TypeScript, the scene compiler generates a field declaration for the list:
private pickableItems: Array<Melon|Bomb>;
Note that the scene compiler infers the type of the array elements: a union of Melon
and Bomb
, that are prefabs. It also infers the type of the elements if are Phaser built-in types:
private parallax: Phaser.GameObjects.Image[];
This detailed type declaration of the arrays allows that code editors like Visual Studio Code can provide a smarter coding experience.
Object List properties
The Object List type is not part of the Phaser API, it is something introduced by the Scene Editor. It has the Variable properties and it also shows the List section in the Inspector view.
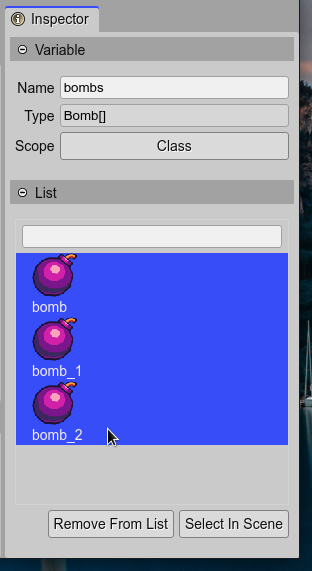
The Remove From List and Select In Scene buttons apply to the items selected in the viewer next to them.
Object List vs Phaser Group
Traditionally, Phaser uses the Group game object to join objects around a common purpose. But grouping is not the only feature of a Group game object:
It implements an object pool.
Is a data structure with methods for sorting and iterate the items.
Can be used as a proxy to modify the state of the items.
To call a method of the items.
To apply game-related global operations to the items.
So, why the Scene Editor is not using the Group game object?
Many of the features of a Group game object are especially helpful to implement the logic of the game, but what a level maker needs is just to organize the objects, we think.
The Group game object type is not parameterized. A code editor type-inference/auto-completion engine works much better with a simple JavaScript array. When you write
const enemies = [enemy1, enemy2, ...]
, a code editor like Visual Studio Code can infer the type of the array items.Even for a human, a simple JavaScript array could be more comprehensible.
If you need a Group game object, you can create it with the array of objects generated by the Scene Editor:
const pickableItemsGroup = this.add.group(this.pickableItems);